Search looks for provided text the Title, Description, Classification, and Keywords for the video.

Properties and Methods
Unlock the power of Properties and Methods in PHP OOP! In this action-packed lesson, we dive into public and private properties & methods, breaking down the magic of encapsulation 🔒. Watch as we create a class, instantiate objects, and interact with public properties & methods from the outside 🌎. But wait—what about private ones? 🤔 No worries! I’ll show you how to work with them inside the class like a pro.
Get ready to level up your PHP skills one method at a time! ⚡👨💻
oop,php
12m:3s
Mar 22, 2025

Publishing Your Package on Packagist
Now it’s time to publish our package and make it available to the world! 🌍 We’ll walk through submitting our package to Packagist and using Composer to install it in a project.
📌 What you’ll learn:
✅ Registering a package on Packagist
✅ Using Composer to install your package
✅ Replacing old code with the new package
By the end of this video, your PHP package will be live and ready for developers to use! 🚀
🔔 Subscribe for more PHP tutorials!
🔗 Resources:
• Packagist Guide
composer,packagist,php
8m:41s
Mar 11, 2025
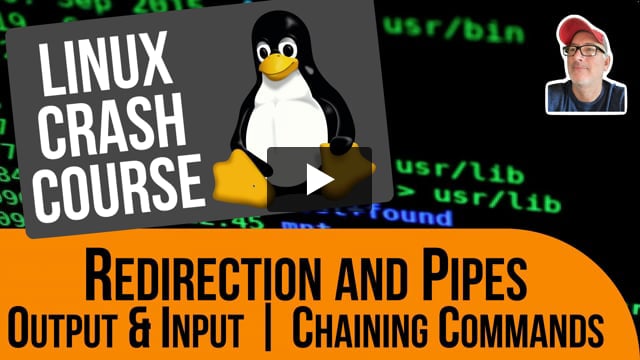
Redirection and Pipes - Output & Input, Chaining Commands
This video is part of the Linux Crash Course series. This video will continue exploring the Linux File System. In this video I will discuss redirecting stdin, stdout, and stderr. In addition, we will look a chaining commands together using pipes.
Github Repo: https://github.com/rcravens/linux-crash-course
linux
32m:13s
Feb 3, 2025

Reusable Code
Writing clean, maintainable code is a key skill for any developer! In this video we take our calculator project to the next level by refactoring it for better structure and reusability.
Here’s what you’ll learn:
✅ Refactoring code – Moving related logic into separate files.
✅ require & include – How to properly import and reuse PHP files.
✅ Why structured code matters – Better organization, easier maintenance, and reusability.
By the end of this tutorial, you’ll have a more structured, modular, and reusable PHP application—just like professional developers do!
php
8m:32s
Mar 19, 2025

Setting Up the Package Structure
In this video, we start coding! 🚀 We’ll create the necessary directory structure, initialize a Git repository, and write the initial code for our PHP package.
📌 What you’ll learn:
✅ Setting up the directory structure
✅ Initializing a Git repository
✅ Writing the first version of the package
💡 By the end of this video, you’ll have a solid foundation for your package!
🔔 Subscribe for more PHP tutorials!
🔗 Resources:
• GitHub: Getting Started
github,package,php
14m:42s
Mar 11, 2025

Setup a Development Environment
Before you start coding in PHP, you need the right tools! In this video, we’ll guide you through setting up a local development environment step by step. You’ll learn how to install PHP, set up a local server using XAMPP, MAMP, or the built-in PHP server, and explore containerized options like Docker. We’ll also discuss different code editors, from lightweight options like Sublime Text to powerful IDEs like PHPStorm and Visual Studio Code. By the end of this video, you’ll have everything configured and ready to start coding in PHP like a pro! 🚀
php
13m:4s
Mar 17, 2025

Static Methods and Properties
Ever wondered when to use static properties and methods? 🤔 In this video, we break it all down! 🔍
We’ll start with a quick refresher on normal properties and methods, then dive into their static counterparts. 🏗️ What’s the difference? Static members belong to the class—shared across all instances—while normal members are instance-specific. 🌎➡️📦
But wait—static properties aren’t constants! ⚠️ We’ll explore constant properties using const and discuss real-world use cases where static methods shine. ✨
By the end, you’ll know exactly when and why to use static members in your PHP projects! 💡💻 Let’s go! 🎬
oop,php
17m:24s
Mar 26, 2025
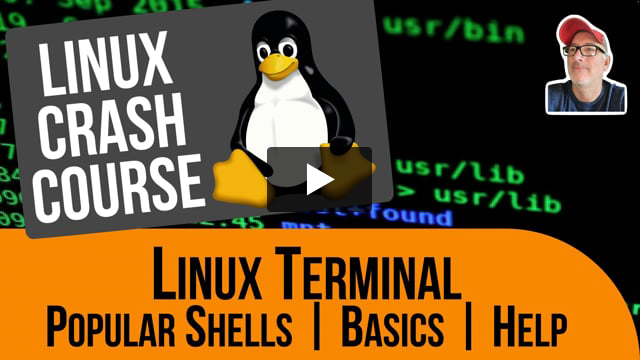
Terminal - Shells, Basics, Help
This video is part of the Linux Crash Course series. This video will introduce the Linux Terminal and how to efficiently navigate command history, cursor position, and how to obtain help on any command.
Github Repo: https://github.com/rcravens/linux-crash-course
linux
12m:34s
Feb 3, 2025
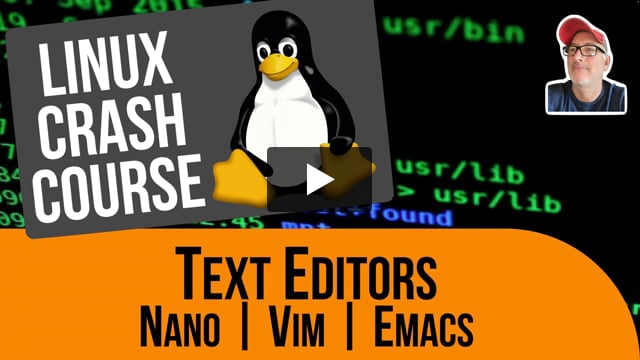
Text Editors - Nano, Vim, Emacs
This video is part of the Linux Crash Course series. This video will continue exploring the Linux File System. In this video I will introduce three common text editors found on Linux systems.
Github Repo: https://github.com/rcravens/linux-crash-course
linux
15m:3s
Feb 3, 2025

Traits
Ever struggled with duplicate code across multiple classes? What if you can’t put it in a base class? 🤔 PHP doesn’t support multiple inheritance, but fear not—Traits come to the rescue! 🦸♂️
In this video, we’ll identify duplicate logic, discuss why it doesn’t belong in the base class, and then refactor using a Trait to keep our code clean, reusable, and DRY! 💡✨
By the end, you’ll be wielding Traits like a pro! 🏆 Let’s dive in! 🎥
oop,php
5m:17s
Mar 25, 2025
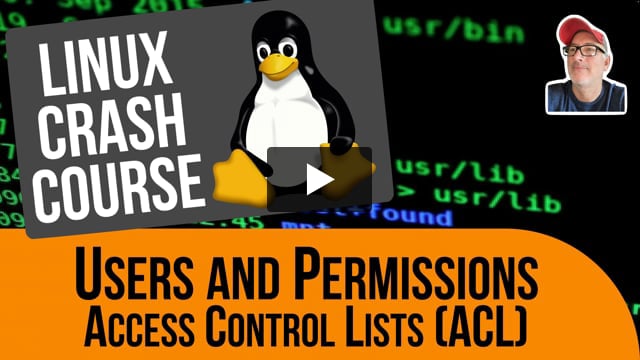
User Permissions - Access Control Lists (ACL)
This video is part of the Linux Crash Course series. This video will cover Access Control Lists or ACLs to apply permissions at a granular level.
Github Repo: https://github.com/rcravens/linux-crash-course
linux
19m:42s
Feb 3, 2025
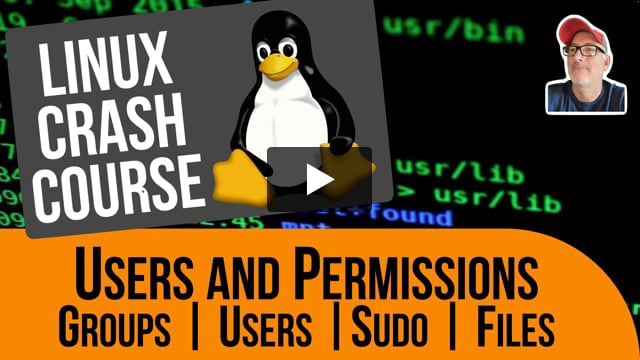
Users and Permissions - Groups, Users, Sudo, Files
This video is part of the Linux Crash Course series. This video will cover user management features.
Github Repo: https://github.com/rcravens/linux-crash-course
linux
1m:45s
Feb 3, 2025

Why OOP?
Ever felt like your PHP code is a tangled mess of functions, impossible to scale, and a nightmare to maintain? You’re not alone! In this kickoff episode, we dive into why Object-Oriented Programming (OOP) is a game-changer and how it solves common problems found in procedural PHP.
We’ll break down the downsides of functional programming—spaghetti code 🍝, duplicated logic 🔁, and function name collisions 💥—and compare it to the structured, reusable, and scalable world of OOP. Through a tabletop comparison, we’ll introduce key OOP concepts like encapsulation, inheritance, and modular design, setting the foundation for everything to come.
By the end of this video, you’ll understand why OOP isn’t just a fancy buzzword—it’s a smarter way to write PHP! 💡🔥
oop,php
13m:52s
Mar 21, 2025

Why PHP?
Kicking off our PHP for Beginners series, this video takes you on a deep dive into the world of PHP. From its origins to its modern-day relevance, we’ll explore how PHP has shaped the web—powering everything from WordPress to robust frameworks and libraries. But why do some developers swear by it while others steer clear? We’ll break down the pros, the cons, and why PHP continues to be a powerhouse in web development today. Whether you’re a complete beginner or just curious about PHP’s place in the tech world, this video is the perfect starting point!
php
8m:11s
Mar 17, 2025

Writing Tests with PEST
Time to make sure our package works as expected! ✅ In this video, we introduce PEST, a powerful and elegant testing framework for PHP. We’ll write automated tests to ensure our package behaves correctly.
📌 What you’ll learn:
✅ Installing and setting up PEST
✅ Writing unit tests for our package
✅ Running and analyzing test results
🚀 Why test? Because a well-tested package is a reliable package!
🔔 Subscribe for more PHP content!
🔗 Resources:
• PEST Documentation
package,pest,php
7m:5s
Mar 11, 2025