Search looks for provided text the Title, Description, Classification, and Keywords for the video.

javascript
12m:42s
Apr 29, 2025
Operators & Expressions
Now that you know how to store data, it’s time to work with it! In this episode, we’ll explore how JavaScript uses operators and expressions to perform calculations, compare values, and make decisions. 🚀🎯 Here’s what we’ll cover:
• Master arithmetic operators like addition, subtraction, multiplication, division, remainder, and exponentiation.
• Use assignment operators to update variable values quickly and efficiently.
• Compare values with comparison operators like ==, ===, !=, <, >, <=, and >=.
• Combine logic with logical operators: and (&&), or (||), and not (!).
🎥 Visual Walkthroughs:
• See real-world examples of manipulating numbers and booleans.
• Watch how combining different operators creates dynamic expressions.
• Practice thinking like a computer: true, false, and everything in between!
By the end of this episode, you’ll be writing powerful expressions and making JavaScript do some real thinking for you! 🧠⚡️

javascript
7m:38s
May 13, 2025
Parameters, Return Values, and Scope
In this episode, we go deeper into how functions communicate — by receiving input, producing output, and knowing where variables “live.” 🧩🎯 Here’s what we’ll cover:
• How to pass data into functions using parameters and arguments.
• Use return values to get results back from functions.
• Understand scope — where your variables exist and who can see them:
• Local (block), function, and global scope.
• Get a taste of lexical scope with a simple nested function.
🎥 Visual Walkthroughs:
• Watch functions take in inputs and return meaningful results.
• See clear, visual examples of variable scope in action.
• Spot common mistakes like trying to access variables outside their scope.
After this episode, you’ll be writing smarter functions that take input, give output, and stay in their own lane! 🧠➡️🧪➡️📦
Coming Soon

oop,php
22m:11s
Jul 8, 2025
Photo Deletion
🖼️ Improved Photo Show PageIn photo.show, hide the summary and reviews if none exist
🔗 Delete Route & Form
Add a new route using the DELETE method
In photo.show, display a delete form only if the logged-in user owns the photo
Include a hidden input field: _METHOD = DELETE
Update the Router class to detect the method via:
$_POST['_METHOD'] ?? $_SERVER['REQUEST_METHOD']
🔧 Controller Refactor
Split responsibilities:
ReviewController: keep only the store method, and fix redirect_back() usage
PhotoController: remove the store method
🧨 Destroy Method in PhotoController
Ensure the user is authenticated
Validate the photo exists
Validate ownership of the photo
Delete the photo file:
Add directory() method to the Photo model
Add convert_to_path() method to the Photo model
Delete the corresponding database record:
Add a delete() method to the base Model class
📌 That wraps up the deletion flow and lands us at v26-delete-photos — now users can clean up after themselves!
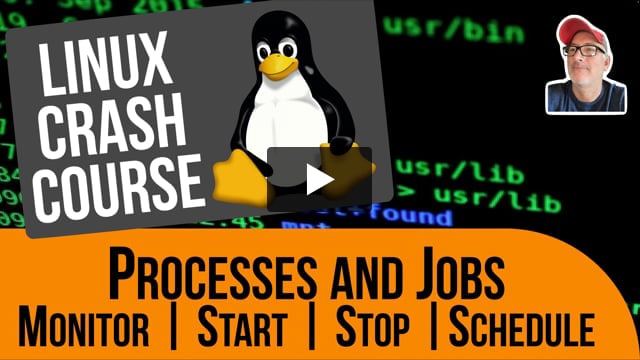
linux
38m:26s
Feb 3, 2025
Processes and Jobs - Monitor, Kill, Background Jobs, Crontab, SystemD
This video is part of the Linux Crash Course series. This video will cover how to manager processes and jobs. We will cover the following:1. How to monitor running processes
2. How to kill or stop existing processes
3. Run a script in the background as a job
4. Run a script at a scheduled interval (e.g., once per minute...) using crontab
5. Run a script as a service using systemd
Github Repo: https://github.com/rcravens/linux-crash-course

oop,php
12m:3s
Mar 22, 2025
Properties and Methods
Unlock the power of Properties and Methods in PHP OOP! In this action-packed lesson, we dive into public and private properties & methods, breaking down the magic of encapsulation 🔒. Watch as we create a class, instantiate objects, and interact with public properties & methods from the outside 🌎. But wait—what about private ones? 🤔 No worries! I’ll show you how to work with them inside the class like a pro.Get ready to level up your PHP skills one method at a time! ⚡👨💻

composer,packagist,php
8m:41s
Mar 11, 2025
Publishing Your Package on Packagist
Now it’s time to publish our package and make it available to the world! 🌍 We’ll walk through submitting our package to Packagist and using Composer to install it in a project.📌 What you’ll learn:
✅ Registering a package on Packagist
✅ Using Composer to install your package
✅ Replacing old code with the new package
By the end of this video, your PHP package will be live and ready for developers to use! 🚀
🔔 Subscribe for more PHP tutorials!
🔗 Resources:
• Packagist Guide
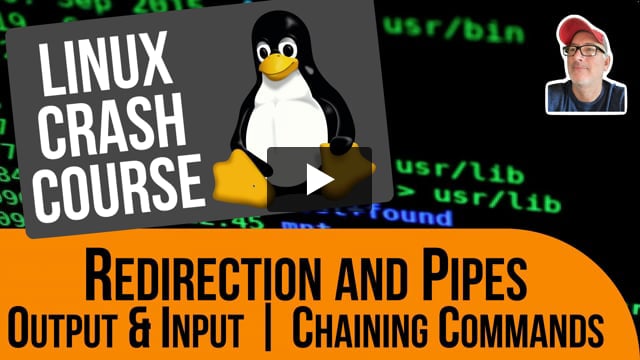
linux
32m:13s
Feb 3, 2025
Redirection and Pipes - Output & Input, Chaining Commands
This video is part of the Linux Crash Course series. This video will continue exploring the Linux File System. In this video I will discuss redirecting stdin, stdout, and stderr. In addition, we will look a chaining commands together using pipes.Github Repo: https://github.com/rcravens/linux-crash-course

php
8m:32s
Mar 19, 2025
Reusable Code
Writing clean, maintainable code is a key skill for any developer! In this video we take our calculator project to the next level by refactoring it for better structure and reusability.Here’s what you’ll learn:
✅ Refactoring code – Moving related logic into separate files.
✅ require & include – How to properly import and reuse PHP files.
✅ Why structured code matters – Better organization, easier maintenance, and reusability.
By the end of this tutorial, you’ll have a more structured, modular, and reusable PHP application—just like professional developers do!

oop,php
10m:34s
May 13, 2025
Router Infrastructure
Let’s bring structure and scalability to our routing system! In this video, we encapsulate all routing logic into a dedicated Router infrastructure class, giving us a clean and centralized way to manage routes. 🧭Here’s what we’ll accomplish:
• Move and rename load_route() into the new Router class as a static view() method 🔁
• Add static get() and post() methods to define routes declaratively 🚪
• Simplify how our application connects URLs to controllers using a centralized approach 🧠
By the end, we’ll have version v10-router-infra—a solid foundation for routing that’s clean, powerful, and easy to scale. 🚀
Coming Soon

oop,php
19m:31s
Jul 10, 2025
Second Refactor & Review
🧠 Model Hydration Fixes- Solved the issue where results weren’t instances of the child model class
- Introduced hydrate() and hydrate_rows() to handle model instantiation
- Added PHPDoc annotations with generics to assist with static analysis and better typing
- Updated type hints across Policies and Controllers for clarity and consistency
📦 Model Enhancements
- Added an update() method
- Introduced pagination support with skip() and take()
- Added sorting via order_by() and order_by_desc()
- Modified / route to use pagination
🛡️ New Policy Classes
- PhotoController: created Http/Policies/PhotoPolicy
- AuthController: created and used AuthPolicy
- RegisterController: created UserPolicy
- ReviewController: created ReviewPolicy
- UploadController: updated to use PhotoPolicy
- Fixed return $data in UploadController to include a proper URL
📌 All of that polish and organization lands us at v26-review-refactor — making the codebase smoother, smarter, and more maintainable.

oop,php
25m:6s
Jun 10, 2025
Sessions / Redirects / Flash Messages
Now that users can submit reviews, let’s give them feedback! In this video, we bring in sessions, redirects, and flash messaging to guide the user experience after form submissions. ✨Here’s what gets built in version v18-redirects-with-flash:
- Create a Session class as a singleton, with a handy global session() helper 🔐
- Initialize the session at app start and build a clean API like:
- session()->error('Missing required parameters.')->redirect('/');
- Implement error() and redirect() methods for smooth flow and messaging
- Fix the issue of persistent flash messages by introducing delete_transient_data() to clear session data after one request cycle
- Use Alpine.js to display flash messages dynamically with a snappy user experience ⚡
- Create a reusable _flash.view.php layout file for flash UI 💡
- Hook flash messages into the review flow by updating all dd('TODO') redirects in the PhotoController
- Bonus: Add current_route support in the Router to improve context-awareness in views 🧭
With sessions and flash messages in place, your app now speaks back to the user—clear, friendly, and fast. 🚀

javascript
6m:24s
Apr 17, 2025
Setting Up The Environment
Before we dive deep into coding magic, we need a proper space to create it! In this episode, we’ll set up a clean and powerful JavaScript development environment so you can code confidently from the start. 🛠️✨🚀 Here’s what we’ll cover:
• Introduce two popular code editors: Visual Studio Code and WebStorm. You can choose either one to follow along comfortably!
• I’ll be using WebStorm throughout this series for demonstrations.
• Set up your first project folder called js-beginners, and create two important files inside: index.html and script.js.
• Learn how to open your HTML file in the browser and access the browser developer tools to run and inspect your JavaScript code.
• Connect your external JavaScript file to your HTML page so your scripts come alive right inside the browser console!
🎥 Visual Walkthroughs:
• We’ll create the project structure together, step-by-step.
• Open the browser console and verify everything’s working.
• Highlight important parts of the editor and project setup to keep things clean and organized.
By the end of this episode, you’ll have your environment ready, your first files created, and the confidence to start coding with JavaScript! 🚀

github,package,php
14m:42s
Mar 11, 2025
Setting Up the Package Structure
In this video, we start coding! 🚀 We’ll create the necessary directory structure, initialize a Git repository, and write the initial code for our PHP package.📌 What you’ll learn:
✅ Setting up the directory structure
✅ Initializing a Git repository
✅ Writing the first version of the package
💡 By the end of this video, you’ll have a solid foundation for your package!
🔔 Subscribe for more PHP tutorials!
🔗 Resources:
• GitHub: Getting Started

php
13m:4s
Mar 17, 2025
Setup a Development Environment
Before you start coding in PHP, you need the right tools! In this video, we’ll guide you through setting up a local development environment step by step. You’ll learn how to install PHP, set up a local server using XAMPP, MAMP, or the built-in PHP server, and explore containerized options like Docker. We’ll also discuss different code editors, from lightweight options like Sublime Text to powerful IDEs like PHPStorm and Visual Studio Code. By the end of this video, you’ll have everything configured and ready to start coding in PHP like a pro! 🚀
esp32
23m:9s
Apr 4, 2025
Software
Now that the hardware’s wired up, it’s time to breathe life into the Smart Garage Door with code! 🚀In this episode, we walk through the entire software that powers the project — written with clean, object-oriented design to keep things modular and maintainable 🧼👨💻.
We do a full code review and break down the key classes that make everything tick:
🔹 DoorSensor – encapsulates logic for detecting the door’s position
🔹 DoorButton – handles interactions with the door opener button
🔹 GarageDoor – the high-level controller that integrates with Apple Home using HomeSpan. It pulls it all together and exposes the device as a HomeKit accessory 🍏🏠
You’ll see how these components work together — and how dependency injection keeps things clean by passing DoorSensor and DoorButton instances right into the GarageDoor class constructor.
Whether you’re into embedded dev, smart home integrations, or just love clean code, this video dives into the software heart of the project. Let’s build smart! 💡💻